概述
本文讲的是,使用 servlet+jsp+jdbc+mysql 实现一个简单的商品管理系统,不使用任何框架,从而加深自己对 java web 基础知识的理解。
实现的主要功能有:
(1) 对商品信息的增删查改
(2) 用户的登录注册功能
本文主要讲商品信息的增删查改,下一篇文章讲用户的登录注册。
项目结构
项目目录如下:
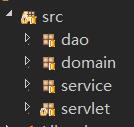
本项目使用 MVC 设计模式(Mode-View-Controller) :
- Controller:负责转发请求,对请求进行处理。本项目使用 servlet 来实现。
- View:负责界面展示。本项目使用 jsp 实现。(本项目 jsp 文件位于 WebContent 目录下)
- Model: 负责操作数据库。本项目 model 包含 domain 包和 dao 包。
此外,项目中还有一个 service 包,负责实现业务逻辑。包与包之间的关系是:
(1) domain 中的一些类映射数据库中的表,
(2) dao 包使用 jdbc 操作数据库,并将结果映射到 domain 中的java bean类,
(3) service 包调用 dao 包的方法来实现业务逻辑,
(4) servlet 包调用 service 包的方法来处理或者转发请求,以及实现页面的跳转。
项目具体文件如下:
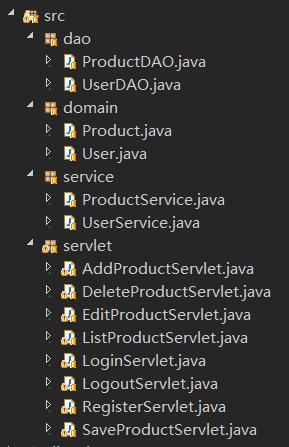
项目实现
数据库建表及插入数据
新建数据库 management,设置编码为 utf-8
1
| create database management character set utf8;
|
创建表 product 并插入数据
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19
| use management; drop table if exists product; create table product( id int auto_increment, name char(30), price float, primary key(id) );
insert into product (name,price) values ('飞机',1000.0); insert into product (name,price) values ('大炮',500.5); insert into product (name,price) values ('子弹',32.0); insert into product (name,price) values ('方舟',700.0); insert into product (name,price) values ('软件',980.0); insert into product (name,price) values ('飞船',60.0); insert into product (name,price) values ('神威',80.6); insert into product (name,price) values ('筷子',78.5); insert into product (name,price) values ('篮球',65.4); insert into product (name,price) values ('平板',54.7);
|
domain 包
domain 包下新建 Product 类。该类对应于 product 表,类中的三个属性也对应于 product 表的列名。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26
| package domain;
public class Product { private int id; private String name; private float price;
public int getId() { return id; } public void setId(int id) { this.id = id; } public String getName() { return name; } public void setName(String name) { this.name = name; } public float getPrice() { return price; } public void setPrice(float price) { this.price = price; } }
|
dao 包
DAO(数据库操作对象,Database Access Object)是 JDBC 下常用的模式,保存数据时它获取 java bean 的属性值,插入 sql 语句中,并保存到数据库中;读取数据时将数据从数据库中读取出来,并将值设置到 java bean 的属性中。
本项目与数据库直接相关的操作都放在 dao 包中。在此包下新建 ProductDAO 类,类中有对商品信息进行增删查改的各个方法。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171
| package dao; import domain.Product; import java.sql.*; import java.util.ArrayList; import java.util.List; public class ProductDAO { public Connection getConnection() throws SQLException{ DriverManager.registerDriver(new com.mysql.jdbc.Driver()); Connection conn = DriverManager.getConnection( "jdbc:mysql://localhost:3306/management?characterEncoding=utf-8", "root","admin"); return conn; } public int addProduct(Product p){ Connection conn = null; PreparedStatement preStmt = null; int result=0; String sql = "insert into product (name,price) values (?,?)"; try{ conn = getConnection(); preStmt = conn.prepareStatement(sql); preStmt.setString(1, p.getName()); preStmt.setFloat(2, p.getPrice()); result = preStmt.executeUpdate(); if(preStmt!=null) preStmt.close(); if(conn!=null) conn.close(); }catch(SQLException e){ e.printStackTrace(); } return result; } public int deleteProduct(int id){ Connection conn = null; PreparedStatement preStmt = null; int result=0; String sql = "delete from product where id=?"; try{ conn = getConnection(); preStmt = conn.prepareStatement(sql); preStmt.setInt(1, id); result = preStmt.executeUpdate(); if(preStmt!=null) preStmt.close(); if(conn!=null) conn.close(); }catch(SQLException e){ e.printStackTrace(); } return result; } public int updateProduct(Product p){ Connection conn = null; PreparedStatement preStmt = null; int result=0; String sql = "update product set name=?,price=? where id=?"; try{ conn = getConnection(); preStmt = conn.prepareStatement(sql); preStmt.setString(1, p.getName()); preStmt.setFloat(2, p.getPrice()); preStmt.setInt(3, p.getId()); result = preStmt.executeUpdate(); if(preStmt!=null) preStmt.close(); if(conn!=null) conn.close(); }catch(SQLException e){ e.printStackTrace(); } return result; } public List<Product> listProduct(){ Connection conn = null; PreparedStatement preStmt = null; ResultSet rs = null; String sql = "select * from product"; List<Product> plist=new ArrayList<>(); try{ conn = getConnection(); preStmt = conn.prepareStatement(sql); rs = preStmt.executeQuery(); while(rs.next()){ Product p=new Product(); p.setId(rs.getInt("id")); p.setName(rs.getString("name")); p.setPrice(rs.getFloat("price")); plist.add(p); } if(preStmt!=null) preStmt.close(); if(conn!=null) conn.close(); }catch(SQLException e){ e.printStackTrace(); } return plist; } public Product findProductById(int id){ Connection conn = null; PreparedStatement preStmt = null; ResultSet rs = null; String sql = "select * from product where id=?"; Product p=new Product(); try{ conn = getConnection(); preStmt = conn.prepareStatement(sql); preStmt.setInt(1, id); rs = preStmt.executeQuery(); if(rs.next()){ p.setId(rs.getInt("id")); p.setName(rs.getString("name")); p.setPrice(rs.getFloat("price")); } if(preStmt!=null) preStmt.close(); if(conn!=null) conn.close(); }catch(SQLException e){ e.printStackTrace(); } return p; } }
|
service 包
service 包主要负责业务逻辑的实现,本项目比较简单,只实现商品信息的增删查改,所以只是简单调用了 dao 包中的方法。新建 ProductService 类。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29
| package service; import domain.Product; import java.util.List; import dao.ProductDAO; public class ProductService { private ProductDAO pdao = new ProductDAO(); public int addProduct(Product p){ return pdao.addProduct(p); } public int deleteProduct(int id){ return pdao.deleteProduct(id); } public int updateProduct(Product p){ return pdao.updateProduct(p); } public List<Product> listProduct(){ return pdao.listProduct(); } public Product findProductById(int id){ return pdao.findProductById(id); } }
|
servlet 包
新建 ListProductServlet 类,调用 ProductService 类中的 listProduct()
方法获取 product list ,并将此传递到 listProduct.jsp
界面,来展示所有商品。ListProductServlet.java
主要代码如下:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15
| package servlet;
@WebServlet("/listProduct") public class ListProductServlet extends HttpServlet{ private ProductService ps = new ProductService(); @Override public void service(HttpServletRequest request,HttpServletResponse response) throws ServletException,IOException{ List<Product> plist = ps.listProduct(); request.setAttribute("plist", plist); request.getRequestDispatcher("listProduct.jsp").forward(request, response); } }
|
listProduct.jsp
代码如下(注意编码设置为 utf-8):
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48
| <%@ page language="java" contentType="text/html; charset=utf-8" pageEncoding="utf-8"%> <%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %> <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html> <head> <meta http-equiv="Content-Type" content="text/html; charset=utf-8"> <title>Insert title here</title> </head> <body> <table align='center' border='1' cellpadding='4'> <tr > <td>id</td> <td>name</td> <td>price</td> <td colspan='2'>操作</td> </tr> <c:forEach items="${plist}" var="product"> <tr> <td>${product.id }</td> <td>${product.name }</td> <td>${product.price }</td> <td><a href='deleteProduct?id=${product.id }'>删除</a></td> <td><a href='editProduct?id=${product.id }'>编辑</a></td> </tr> </c:forEach> </table> <br><br>
<form action="addProduct" method='post'> <table align='center'> <tr> <td>name</td> <td><input type='text' name='name' value='${product.name }'></td> </tr> <tr> <td>price</td> <td><input type='text' name='price' value='${product.price }'></td> </tr> <tr> <td><input type='submit' value='增加商品' ></td> </tr> </table> </form> </body> </html>
|
此界面中,还包含删除商品,编辑商品以及增加商品的链接或者按钮。摘取如下:
1 2 3
| <td><a href='deleteProduct?id=${product.id }'>删除</a></td> <td><a href='editProduct?id=${product.id }'>编辑</a></td> <form action="addProduct" method='post'>
|
本项目中,是根据商品 id 来删除商品。
DeleteProductServlet.java
主要代码如下:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15
| package servlet;
@WebServlet("/deleteProduct") public class DeleteProductServlet extends HttpServlet{
private ProductService ps = new ProductService();
public void service(HttpServletRequest request,HttpServletResponse response) throws ServletException,IOException{ ps.deleteProduct(Integer.parseInt(request.getParameter("id"))); response.sendRedirect("listProduct"); } }
|
listProduct.jsp
中除了展示所有商品,还包含有用于增加商品的表单,以此提交新增商品的信息,表单中action="addProduct"
,即是 AddProductServlet
的 url-pattern
AddProductServlet.java
主要代码如下:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25
| package servlet;
@WebServlet("/addProduct") public class AddProductServlet extends HttpServlet{
private ProductService ps = new ProductService(); public void service(HttpServletRequest request,HttpServletResponse response) throws ServletException,IOException{ request.setCharacterEncoding("utf-8"); String name = request.getParameter("name"); float price = Float.parseFloat(request.getParameter("price")); Product p = new Product(); p.setName(name); p.setPrice(price); ps.addProduct(p); response.sendRedirect("listProduct"); } }
|
编辑商品信息:在listProduct.jsp
界面中点击“编辑”链接,把商品 id 传递到 EditProductServlet
,EditProductServlet
根据商品 id 查找对应商品的旧信息,然后把旧的信息传递给editProduct.jsp
界面显示出来。接着,在修改界面修改信息后,提交给SaveProductServlet
。最后,在SaveProductServlet
中,调用ProductService
类中的方法更新商品,再跳转到listProduct.jsp
界面。
EditProductServlet.java
主要代码:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17
| package servlet
@WebServlet("/editProduct") public class EditProductServlet extends HttpServlet{
private ProductService ps = new ProductService(); public void service(HttpServletRequest request,HttpServletResponse response) throws ServletException,IOException{ int id = Integer.parseInt(request.getParameter("id")); Product p = ps.findProductById(id); request.setAttribute("product", p); request.getRequestDispatcher("editProduct.jsp").forward(request, response); } }
|
editProduct.jsp
主要代码:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20
| <form action="saveProduct" method='post'> <table align='center'> <tr> <td>name</td> <td><input type='text' name='name' value='${product.name }'></td> </tr> <tr> <td>price</td> <td><input type='text' name='price' value='${product.price }'></td> </tr> <tr> <td><input type='submit' value='保存' ></td> <td><input type='button' value='返回' onclick="location.href='listProduct'"></td> </tr> <tr> <td><input type='hidden' name='id' value='${product.id }'></td> </tr> </table> </form>
|
SavaProductServlet.java
主要代码:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24
| @WebServlet("/saveProduct") public class SaveProductServlet extends HttpServlet{
private ProductService ps = new ProductService(); public void service(HttpServletRequest request,HttpServletResponse response) throws ServletException,IOException{ request.setCharacterEncoding("utf-8"); int id = Integer.parseInt(request.getParameter("id")); String name = request.getParameter("name"); float price = Float.parseFloat(request.getParameter("price")); Product p = new Product(); p.setId(id); p.setName(name); p.setPrice(price); ps.updateProduct(p); response.sendRedirect("listProduct"); } }
|
至此,实现商品信息的增删查改相关代码已编写完毕。输入 http://localhost:8080/Management/listProduct 即可查看商品信息。
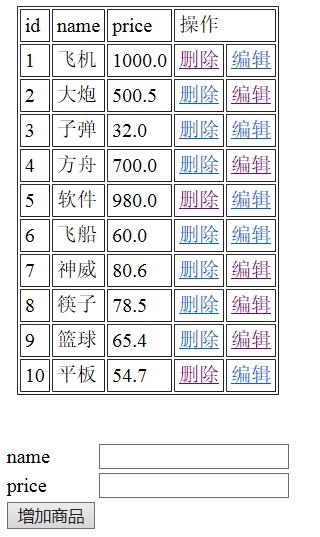
下一篇文章将写用户的登录注册部分
**本文章完整代码见 [Github](https://github.com/anye137/Java-Web-Learning/tree/master/Management)**</font>。